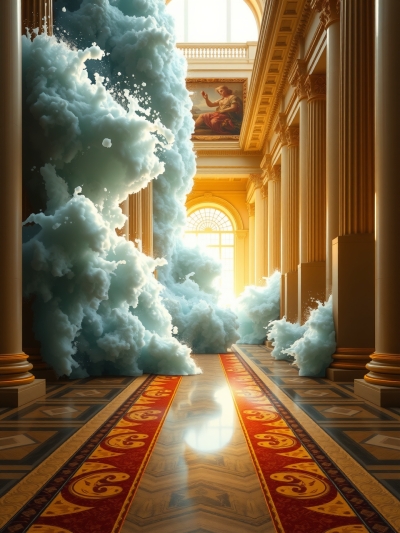
Step 1: Setting Up Your Environment
Before writing PHP code, you need a working environment. You can install a local server like XAMPP, WAMP, or MAMP to run PHP on your computer. These tools provide an Apache server, MySQL database, and PHP interpreter in one package. Alternatively, if you're using Linux, you can set up PHP and Apache manually.
Step 2: Writing Your First PHP Script
A PHP file typically ends with .php and contains PHP code within <?php and ?> tags. Here’s a simple example:
<?php
echo "Hello, World!";
?>
Save this as index.php and open it in your browser via your local server (e.g., http://localhost/index.php). You should see &amp;quot;Hello, World!&amp;quot; displayed on the screen.
Step 3: Learning the Basics
To master PHP, focus on the following core concepts:
Data Types: Strings, integers, floats, booleans, arrays, and objects.
Control Structures: if statements, loops (for, while), and switch.
Functions: Creating reusable blocks of code.
Form Handling: Processing user input from HTML forms.
Database Integration: Using MySQL with PHP (mysqli or PDO) to store and retrieve data.
Step 4: Building Small Projects
The best way to learn PHP is by building projects. Start with simple projects like a contact form, a login system, or a basic CRUD (Create, Read, Update, Delete) application with MySQL. As you progress, explore object-oriented programming (OOP), security best practices, and frameworks like Laravel to enhance your skills.
By following these steps and practicing consistently, you’ll become proficient in PHP in no time. Happy coding! 🚀
Comments (1)